Hello world,
in this blog post, I would like to show you how you can implement the AEP web SDK but without the Launch Extension.
I will still use Launch for the implementation, because you will always use a Tag Manager anyway, but this time, we will use only the CORE – custom code option to implement that. (I know that Jan… )
I already did a blog post on the implementation with the Launch Extension, you can find it here.
Because I already covered some steps in that previous article, and they will remain the same with pure-javascript implementation, I will not cover the following elements:
- Schema creation
- Dataset creation
- Creating your sourceConnection
- Generate your Edge Config ID
You can read that article to get inform about that.
(I worked in SEO, so I know the duplicate content problem 😀 )
Loading the AEP Web SDK
First of all, in order to use the AEP Web SDK library, you will need to load it first with your tag manager.
Several options are possible:
- Download the full library (not recommended)
- Use the CDN option (recommended)
- Install directly on the website via NPM package installation (advanced stuff if you want to ingest that in your website library structure)
You can have all of these options (+ Launch extension of course) described here.
In my case, I will take the CDN option because it is the fastest and I don’t see a real value of hosting the complete JS file on my Lauch implementation, increasing my Launch library size.
I will first load that library THEN (and this word is important) I will configure it.
So the setup looks like this for the configuration at the top of the page.
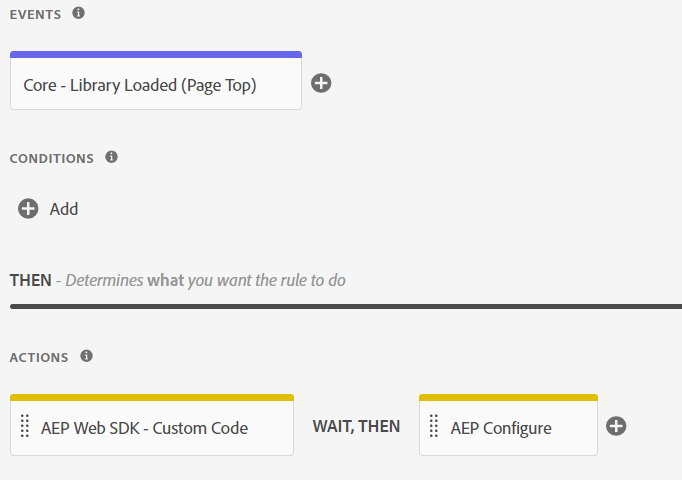
The AEP Web SDK Custom Code will possess this code (in HTML):
The AEP Configure will have this one (in JS):
alloy("configure", {
"edgeConfigId": "0cf39d6b-4ac2-4443-bcdd-08feeee02623",
"orgId":"CA590B1B5D783C2A0A495E47@AdobeOrg",
"defaultConsent":"in"
});
You can see that I have set the rule sequencing again, that is important because you would need to ensure alloy is set* before configuring it.
*set means downloaded and executed.
Setting up Data Elements
During my last article about using the extension, I went very briefly about the Data Elements. Trying not to create an article that was too long to read.
The most important point I tried to convey is that you cannot send another data type than the one expected by your schema.
If you have set your field in your schema to receive strings, or integers, you cannot send a null or undefined type. That is a problem challenge and that is the reason why you would need to setup different XDM schema depending on the the information that you have on your current page.
In my case, I made the choice to set empty string whenever I do not have a value to send. Later on, in AEP CJA, I will be able to override that (future article on CJA).
However, that will require a lot more consideration whenever I use SQL on my datasets with Query Service.
Therefore, you need to rightly chose the way you want to handle missing values. It unfortunately brings an additional layer of complexity in your implementation but trust me that you better spend few more hours defining the data model represented in your dataset, than spending days of handling unexpected elements in your queries.
At the moment, there is a need for quite complex implementation of the AEP Web SDK, I am sure however that additional capabilities will be added later, either on the Web SDK or in the data ingestion process, to improve flexibility and process.
Example of postLength
Sometimes, you have set a field to retrieve a number but you actually need to analyze it as a dimension. That is something that you could actually easily do now in the new CJA interface.
In my case, I am calculating the size length (number of characters) from my post.
From that, I could maybe later find out that longer posts are more attractive (or not).
This is also an element that needs to be coordinated between your implementation team and your data architect team. Some assumptions on both parts could lead to some delay or data being harder than necessary to analyze. Platform is already so complex to set up that going for the simplest implementation of the use-cases is important.
In wordpress, in my template, I am able to retrieve that information with the following code:
try {
var myArticle = document.querySelector("article");
return String(myArticle.innerText.length)
}
catch(e){
return "0"
}
Creating your XDM schema
Once, I have prepared my Data Element, I can actually create my XDM schema and attached these elements to it.
You need to remember that even if you have setup your Launch property to have rule sequencing, Data Elements do not support promise yet.
The implication is that your XDM schema data element should be able to run synchronously and you should not try to retrieve the ECID from the AEP Web SDK in a dimension. It will most likely be possible to do that in the future, but it is not a recommended practice at the moment. The ECID will be automatically collected in the Identity Map. We will see how we can retrieve that when we will see AEP Query Service in a next article.
Because I am using the pure JavaScript implementation, I do not have any helper function here, but if you really understand the AEP data model, it is not that hard to create the skeleton for your AEP Web SDK body message.
In my case, it will look something like this:
value = {
"_emeaconsulting":{
"datanalyst":{
"pageCategory":_satellite.getVar("category"),
"pageSubCategory" : _satellite.getVar("subCategory"),
"postLength" : _satellite.getVar("postLength"),
"postTitle" : _satellite.getVar("pageTitle"),
"returningVisitor" : _satellite.getVar("returningVisitor"),
"searchKeyword" : _satellite.getVar("searchKeyword"),
}
},
"marketing": {
"trackingCode": _satellite.getVar("trackingCode")
}
}
return JSON.parse(JSON.stringify(value));
Note that I stringify this before returning it.
AEP Web SDK always stringify your message before sending it, so your Integers, float or boolean are actually casted during ingestion process.
One could actually think of an extension to actually just return that and remove the elements that have empty string value. This may be the functionality that will come and avoid us from doing complex implementation with multiple XDM body message ready.
Firing Page View rule
Once I get everything up and runnin, I can start to create my Page view rule in Launch. I take the opportunity to add a link to a nice post from Frederik Werner, on how to set up automatic tracking, especially for clicks, and with an event driven data layer, ACDL in his example. It’s worth a read for techies.
In my case, I will use the CORE library to add a custom code:
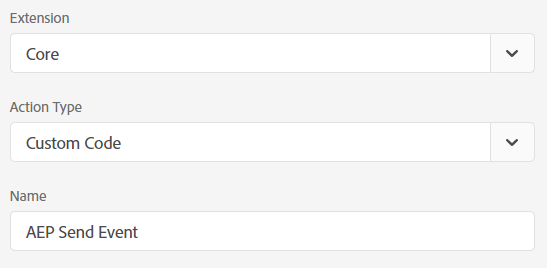
It is important to notice that because it is executed on window.loaded event and it is integrated via the CORE extension, it is not directly integrated into the main launch library. This is a well-known fact from Launch implementation manager, but I still see many people discovering this after too much time. The reason for this is that you do not actively load the script that you do not run on your website. I have done an article, long ago, about the things you should know abour Adobe Launch if you want to know other things like this.
Looking in the JS file uploaded on my website from Launch.
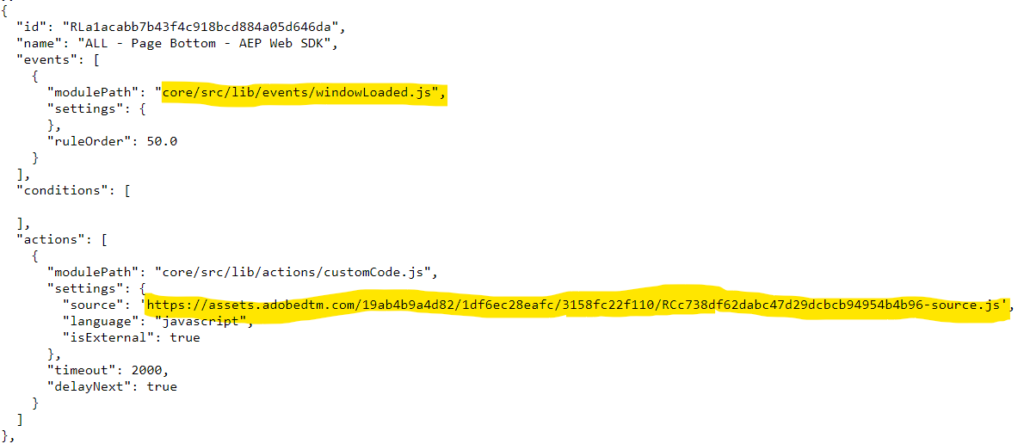
My code inside my rule is pretty straightforward, You just need to fetch the data element containing your XDM body message and attach it to the “xdm” key of your overall object that you are going to send with Alloy / AEP Web SDK.
var myXDMData =_satellite.getVar('xdmPageView')
alloy("sendEvent", {
"xdm": myXDMData,
"type": "web.webpagedetails.pageViews "
});
And if you are on my website right now, because you are reading my article, I encourage you to open the console and check how the data element is formatted.
You should see something similar to this:

I hope this article was helpful on getting to know the JS implementation of the AEP Web SDK for Launch.
This kind of implementation may be required if you are setting the AEP Web SDK in a Launch property inside from organization A and you want to send the data to a different organization (say B) AEP instance.
The AEP Web SDK Extension is currently working when you have AEP and AEP Web SDK in the same organization.
Like the last time, if you want to have a look on how you could Q/A your AEP Web SDK implementation, you can have a look at my other article about this: Validating your AEP Web SDK implementation.