This article will focus on developing a module that will define the firing of the rule or not. It can be managed by the Event module in Launch.
I will first explain how Events are working and show you how I set mine in my module.
You can find the official documentation here :
https://developer.adobelaunch.com/extensions/reference/event-types/
Events in Launch
On Adobe Launch, you need an event in order to fire the rule that you have created. Even for your extensions, you would need to have a rule that contains an event in order to fire them. They are not automatically fired as it used to be for Analytics in DTM.
It is the first thing that you are going to set within Adobe Launch.
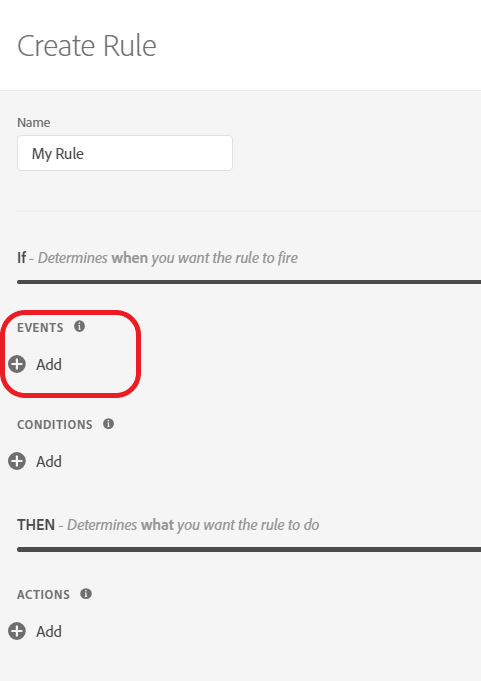
You usually will be using the predefined events that are available from the Core module, but it may interesting for some reasons that you would like to develop your own event.
Because you want to fire some rules based on specific events, that you have developed, you may want to use a custom extension to listen automatically to these type of event.
Event and Condition are different
It sounds pretty dumb to write that events and conditions are different but they are so similar that it is important to notice the difference.
The event will fire the execution of the rule. The condition will be triggered by this event and will evaluate if the rule is allowed to be fired or not.
If you create a rule with the condition “path without query parameters == ‘/example.html’ “. It will not fire on that page if this is what you have set. It requires an event to fire that rule.
Predefined Core Events
As explained briefly, the core module provide predefined events that you can use.
The most notorious ones are:
- DOM Ready : Will trigger when the DOM is ready
- Library Loaded : Will trigger when the library is loaded, it is usually set in the Header, so it should be call at that moment. (be careful for async implementation)
- Page Bottom : It will fire when the pageBottom tag is reached. It works with synchronous implementation.
- Window Loaded : This is trigger when the loadEventEnd is reached on the page.
- Direct Call : This is the infamous Direct Call Rule event that you had with DTM.
- History Change : It will trigger every time the URL change, very useful for Single Page Application.
- Click : The famous click event listener. Notice that there is a hover and mousedown as well.
- Custom Event : It will listen to a specific event that you have set.
- Media : Lots of media possibilities (play, pause, end, volume change, etc…)
- Form : lots of possibilities as well (submit, blur, etc…)
Requirement for Events
The event script will require you to return a trigger function from your module. This is pretty simple. I won’t use it but there is also a possibility to send additional information within your event.
In order to realize that, you would need the trigger function to contain that object.
trigger({nativeEvent: nativeEvent // the value would be the underlying native event });
Adobe recommend you to use the nativeEvent, in case you are listening to a bunch of different events in your event module and they are all related to native events.
Also using the element
if you are connecting the event to a specific element of your page.
Reminder : Documentation from Adobe here.
Event HTML
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Event</title>
</head>
<body>
<h1>My Event Configuration</h1>
<p>This event is going to listen to this type of event: </p>
<input id='event'></input> <button id='default' onClick="default_val()">Default Value</button>
<p>Please replace if you want something different</p>
<script src="https://assets.adobedtm.com/activation/reactor/extensionbridge/extensionbridge.min.js"></script>
<script>
window.extensionBridge.register({
init: function(info) {/*this is for saved setting in your extension - info being the variable passed*/
if(info.extensionSettings){/*in case we want to use the global extension*/
if(info.extensionSettings.event){/*making sure that the event has been configured*/
document.querySelector("#event").value = info.extensionSettings.event || "";
}
}
else if(info.settings.event && info.settings.event!=""){/*if the value has been overwritten by something else in the module*/
document.querySelector("#event").value = info.settings.event;
}
else{/*default is nothing*/
document.querySelector("#event").value = '--nothing--';
}
window.default_val = function(){/*will set the default to the extension setting, or to nothing*/
document.querySelector("#event").value = info.extensionSettings.event || "--nothing--";
// TODO Populate form values from persisted settings.
}
},
getSettings: function() {
myObject = new Object();/*setting a new object*/
myObject['event']=document.querySelector("#event").value;
return myObject;/*return the object*/
},
validate: function() {/*you can return false after some condition to check the correct information has been provided*/
return true
// TODO Return whether settings are valid.
}
});
</script>
</body>
</html>
Event JS
The javascript part that you will run within your website need to return the trigger function at some point, if you want the event to fire.
'use strict';
module.exports = function (settings, trigger) {
var myEventConfig;
myEventConfig = turbine.getExtensionSettings().event;/* retrieve my configuration for my event listening */
window.addEventListener(myEventConfig, function (elem) {
trigger({ element: elem })/* passing the element that trigger the event*/
})
};
I hope that this article helped you to better understand how to create event module within the Launch Extension creation.
On this series, you may be interested by these articles:
Article | Content |
Introdcution to Adobe Launch & Extension Creation | This posts covers the basic of what you need to know in order to start with this series of articles. |
Architecture and dependencies within modules | This posts covers the architectures and how the different modules are linked between each others |
Global Extension Configuration | This article explains how to set up your configuration HTML file. It is the core configuration module of your extension. |
Event type module | This article will show you how to build an event module. Every module will have a configuration part (HTML) and wrapper(JS). |
Condition module | This article will show you how to build a condition module. |
Action module | This post will be related to the action module and how to build it. We will also see how you can actually import some cool feature of the launch library. |
Data Element module | This article will be developing the possibilities of the Data Element module |
Shared module | This article will focus on the shared functionality that you can write and share with other extension developpers to used. We will also used a feature shared from Adobe Analytics to show you how to use it. |
Other features | The other features that are related to Launch Extension. I will cover additional (and important) information there. |
Testing your extension | How do I debug my Extension ? |
Releasing and updating your Extension | How do I publish my Extension into Launch ? And after, how do you update it ? |