On this article, I will focus on explaining the action module, and how to create actions module within your own Adobe Launch Extension.
Actions in Launch
Action are the actual code deliver by the Tag Management system and it will perform once the event has been triggered and the condition is fulfilled.
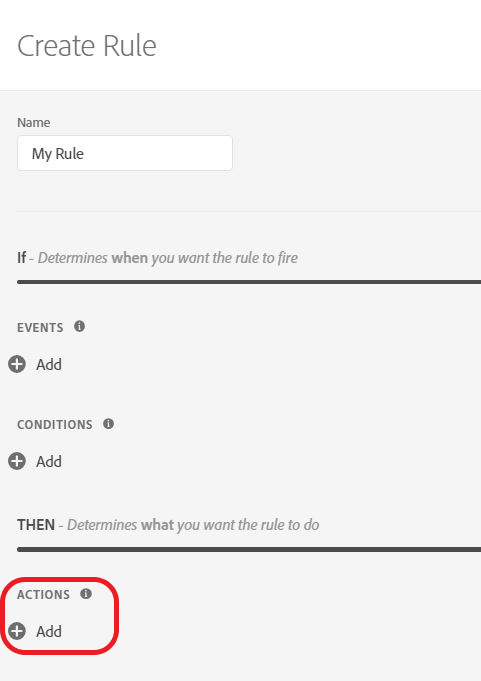
An action can be anything that can be trigger. Usually, as you are writing your own extension, you want to minimize the possibility for your end user to write code.
it is important to notice that you can use any information that you have passed during the writing of your event module.
The official documentation on action can be found here :
https://developer.adobelaunch.com/extensions/reference/action-types/
Action HTML
On the configuration of my action, there is nothing to really configure. I am just showing the actual configuration established in my extension global configuration.
On top of that, I use one of the the turbine view to create a note functionality.
You can save some comment or information in your module by doing so.
Of course, that can also be used to generate code.
You have some view information here.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Action</title>
</head>
<body>
<h1>Action Template</h1>
<p>This is going to be template for my Action. However there is nothing to set here because it has been set in the Extension configuration.</p>
<p>The console would be display : <span id='consoleBool'></span></p>
<p>If set to true, a console.log will print the rule name and rule id</p>
<p>You can set comment for this action by clicking on the button <button id='note' class="ValidationWrapper">Note</button></p>
<script src="https://assets.adobedtm.com/activation/reactor/extensionbridge/extensionbridge.min.js"></script>
<script>
window.myObj = {'comment':''};/*creating the object here, in order to communicate with it later*/
window.code = '';/*default value for opening text editor*/
window.extensionBridge.register({
init: function(info) {
var el = document.querySelector('#consoleBool');
if (info.settings) {
/*nothing can be set by the action configuration - so nothing should happen*/
window.myObj = info.settings /*re-set the code saved*/
console.log(myObj)
window.code = window.myObj.comment;/*expose the previous code*/
}
if(info.extensionSettings.condition){/*take Extension configuration - if any*/
el.innerText += info.extensionSettings.action; /*add the info*/
}
else{
el.innerText += "false";
}
},
getSettings: function() {
/*I would have needed to return,through an object, any info that are setup in the action module configuration.
But I don't set anything, so here it is empty and doing nothing.
*/
myObj = window.myObj;/*retrieve what has been set and keep it as */
return myObj;
},
validate: function() {
return true; /*you can return false after some condition to check the correct information has been provided*/
}
});
</script>
<script>
/*Async Function that populate my object*/
console.log('status on load time : '+window.code);/*in order to see the race condition issue*/
note = document.getElementById('note');
note.addEventListener('click',function(){
code = window.myObj.comment;/*retrieve the actual value passed before - init function has run*/
console.log('status on click : '+code)
var options = {'code':code, 'language':'plaintext'}/*options object to pass the correct setting to my view*/
window.extensionBridge.openCodeEditor(options)
.then(function(code) {
window.myObj['comment'] = code;/*passing the code to my object*/
console.log('code : ' + code);})
});
</script>
</body>
</html>
Action JavaScript
On the code below, I set the action to call the rule name and rule id and display it on the console.
'use strict';
module.exports = function (settings,event) {/*do not forget to add event*/
var myGlobalSetting; /*we have to declare all of the variables because of strict mode*/
myGlobalSetting = turbine.getExtensionSettings().action;/*retrieving the global config*/
if (myGlobalSetting == true) {/*If it is true*/
console.log('Rule ID : ' + event.$rule.id);
console.log('Rule Name : ' + event.$rule.name);
}
else {
turbine.logger.info('Rule ID : ' + event.$rule.id);
turbine.logger.info('Rule Name : ' + event.$rule.name);
}
};
I hope that this article helped you to understand the possibilities of action modules and how to implement them within Adobe Launch.
On this series, you may want to read as well:
Article | Content |
Introdcution to Adobe Launch & Extension Creation | This posts covers the basic of what you need to know in order to start with this series of articles. |
Architecture and dependencies within modules | This posts covers the architectures and how the different modules are linked between each others |
Global Extension Configuration | This article explains how to set up your configuration HTML file. It is the core configuration module of your extension. |
Event type module | This article will show you how to build an event module. Every module will have a configuration part (HTML) and wrapper(JS). |
Condition module | This article will show you how to build a condition module. |
Action module | This post will be related to the action module and how to build it. We will also see how you can actually import some cool feature of the launch library. |
Data Element module | This article will be developing the possibilities of the Data Element module |
Shared module | This article will focus on the shared functionality that you can write and share with other extension developpers to used. We will also used a feature shared from Adobe Analytics to show you how to use it. |
Other features | The other features that are related to Launch Extension. I will cover additional (and important) information there. |
Testing your extension | How do I debug my Extension ? |
Releasing and updating your Extension | How do I publish my Extension into Launch ? And after, how do you update it ? |