On this article I will go through the first steps that you would need to take in order to use the python wrapper for the Adobe Analytics API 2.0.
As I was explaining in the Introduction article, you would need to have an Adobe IO account registered in order to use the python wrapper. Other articles on my blog exist in order to realize that, I let you read them if you didn’t have.
Also be sure to connect the adobe IO account to the correct product profile. You need all access to use all methods.
Installing the python module
At the moment I didn’t create a pypi module that you can easily install from pip so you would need to install that module manually.
What I mean by that, is that you can download the .py module and place it in your python repository.
You can find the module here : https://github.com/pitchmuc/adobe_analytics_api_2.0
What I do, in order to ensure that the package is accessible by python, is to place to the python3X/Lib folder.
By doing that, you can import it from anywhere as you normally have added the python folder in the window environment path.
Setting the config file
Once you have imported the module into your work, the first thing to do is to create / generate a config file.
The config file is a JSON file that encapsulate all the information that are needed for your API connection to work. It looks like this :
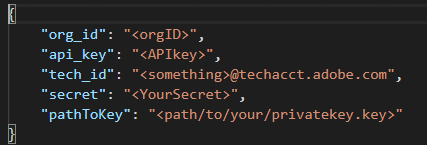
The good news is that you don’t need to setup the config file every time, you modify it, you save it, and then you will be able to use it directly next time.
There is a specific method that you should always run when you start with the module, it is the importConfigFile method, and it sets up everything, according to your config file.
Note : You can see that there is a pathToKey element, you would need to have the path to the key set here, it can be quite complicated and can create error quite easily because the pathing system is not the same on Linux, Mac and Windows.
There are way to go around this but I didn’t implement them, so I would recommend you to put the privatekey unencoded in the same folder than the one used for Analytics API connections.
At the end of that section, you file should looks like :
import aanalytics2 as api2 ##just for sake of shorting it
api2.createConfigFile()
### then when the config file is set
api2.importConfigFile('config_analytics_api.json')
Looking for company ID
Adobe Analytics API 2.0 does a very particular thing with the API connection, it is that you would need to pick the company ID that you want and then use it within the header of your requests.
This doesn’t seem a big deal but it is quite annoying that you should change the header of your request based on your company ID. It is unusual and cumbersome. In our case, it involves an extra step.
Company ID
First you would need to set your company ID.
You can do that by using the getCompanyId method and it will return you a list of dictionary.
officially it was a bit more complicated than that, but I assumed that everyone can select the correct company ID with the information I provide. The response usually looks like this :
[{'globalCompanyId': 'cid1',
'companyName': 'Company One',
'apiRateLimitPolicy': 'aa_api_tier10_tp',
'dpc': 'lon'},
{'globalCompanyId': 'cid2',
'companyName': 'Company two',
'apiRateLimitPolicy': 'aa_api_tier30_tp',
'dpc': 'lon'}]
You would need to select then the correct “globalCompanyId” and save it in a variable. You would need to use it later on.
Updating the Header #DEPRECATED
The next step is the extra step that I told you about, you would need to update the header with the “globalCompanyId” you have selected.
There is a method for that : updateHeader
So you would need to run this method before going on the next step.
At the end of that step, your file/notebook should look like this :
cids = api2.getCompanyId()
cid = cids[0]['globalCompanyId']
api2.updateHeader(cid)
Instantiate the Analytics class
Since May 2020, the Analytics class needs to be instantiated in order to call the other methods discussed in the following articles.
import aanalytics2 as api2 api2.importConfigFile('myconfig.json') cids = api2.getCompanyId() cid = cids[0]['globalCompanyId'] ## using the first one mycompany = api2.Analytics(cid)
This will enable you to connect to several login companies at the same time by creating several instance of the same class.
Note that a new method is available from the Analytics class. It enable you to replace the token with a new one if your token expire (more than 24h).
It is call refreshToken(token) and token is the string of the token.
May be updated later on to make token argument optional.
Note for single company user
Most of you may have only one company and therefore don’t need to select anything. Therefore this process is quite cumbersome. Also if you already know which company to take, it is quite annoying.
For that reason, I created a shortcut to that process.
When you use the method getCompanyId, you can pass a parameter and this parameter lets you specify if you want to select a specific globalCompanyId and directly update the header for it.
You pass a number as string but you can also pass “first” to take the first globalCompanyId.
In that case, your process would look like :
cid = api2.getCompanyId(infos='first') ## I want the first one
##or
cid = api2.getCompanyId(infos='0') ## I want the first one
##or
cid = api2.getCompanyId(infos='1') ## I want the 2nd one
Once you have done all of that, you are ready to use the API with all of its functionalities.
Articles | Comments |
Adobe IO : JWT authentication | How to create JWT authentication with OpenSSL and python |
Adobe IO : Analytics API 2.0 : Introduction | What you need to know about this module |
Adobe IO : Analytics API 2.0 : Connection to Analytics | Getting started with the python wrapper. |
Adobe IO : Analytics API 2.0 : Retrieving Elements | How to retrieve evars, metrics, users, segments, etc… |
Adobe IO : Analytics API 2.0 : Reporting | How to get a report from the new Analytics API 2.0 |
Adobe IO : Analytics API 2.0 : Tutorial | Video to demonstrate how to use the Analytics API 2.0 with the python wrapper |
Looks like JWT will be deprecated in favor of OAuth2 libraries, can you confirm if this is on your roadmap?
Yes, it is, it is already taking care of for the aepp library. I need to do a small change in the config loading in order to accomodate for it.